Briefly list the advantages of grpc over traditional restful api
- High performance: Through HTTP/2 and Protobuf, the latency and bandwidth consumption of data transmission are greatly reduced.
- Multiple communication modes: Supports complex communication modes such as bidirectional streaming and unidirectional streaming.
- Cross-language support: Suitable for building cross-language distributed systems.
- Automatic code generation: Automatically generate client and server code based on interface definition to reduce duplication of work.
- Built-in load balancing and security mechanism: Make communication in microservice architecture more efficient, reliable and secure.
gui about grpc
https://github.com/bloomrpc/bloomrpc
just import proto file and the function inside will auto import, just need set params and url,
create two projects in one directory, one as client, the other one as server, create proto to manage common proto file
create proto file
syntax = "proto3";
package person;
// Generated according to https://cloud.google.com/apis/design/standard_methods
service PersonService {
rpc FindOne (PersonById) returns(Person) {}
rpc FindAll (Empty) returns(People) {}
}
message PersonById {
int32 id = 1;
}
message Person {
int32 id = 1;
string name = 2;
string power = 3;
}
message Empty {
}
message People {
repeated Person people = 1;
}
Achieve Server
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
import { join } from 'path';
import { MicroserviceOptions, Transport } from '@nestjs/microservices';
async function bootstrap() {
const app = await NestFactory.create(AppModule);
app.connectMicroservice<MicroserviceOptions>({
transport: Transport.GRPC,
options: {
package: 'person',
protoPath: join(__dirname, '../../proto/person.proto'),
url: 'localhost:5000',
},
});
await app.startAllMicroservices();
await app.init();
}
bootstrap();
Set Script
add running dependency
pnpm add @nestjs/microservices
add dev dependencities
pnpm add ts-proto @grpc/grpc-js @grpc/proto-loader -D
add script in package scripts
"proto:gen": "protoc --plugin=protoc-gen-ts_proto=./node_modules/.bin/protoc-gen-ts_proto --ts_proto_out=./proto/ --ts_proto_opt=nestJs=true src/proto/*.proto"
excute script
pnpm run proto
it will auto generate ts file including grpcmethod decorator of fucntion we wrote
// Code generated by protoc-gen-ts_proto. DO NOT EDIT.
// versions:
// protoc-gen-ts_proto v2.6.1
// protoc v5.28.3
// source: person.proto
/* eslint-disable */
import { GrpcMethod, GrpcStreamMethod } from "@nestjs/microservices";
import { Observable } from "rxjs";
export const protobufPackage = "person";
export interface PersonById {
id: number;
}
export interface Person {
id: number;
name: string;
power: string;
}
export interface Empty {
}
export interface People {
people: Person[];
}
export const PERSON_PACKAGE_NAME = "person";
/** Generated according to https://cloud.google.com/apis/design/standard_methods */
export interface PersonServiceClient {
findOne(request: PersonById): Observable<Person>;
findAll(request: Empty): Observable<People>;
}
/** Generated according to https://cloud.google.com/apis/design/standard_methods */
export interface PersonServiceController {
findOne(request: PersonById): Promise<Person> | Observable<Person> | Person;
findAll(request: Empty): Promise<People> | Observable<People> | People;
}
export function PersonServiceControllerMethods() {
return function (constructor: Function) {
const grpcMethods: string[] = ["findOne", "findAll"];
for (const method of grpcMethods) {
const descriptor: any = Reflect.getOwnPropertyDescriptor(constructor.prototype, method);
GrpcMethod("PersonService", method)(constructor.prototype[method], method, descriptor);
}
const grpcStreamMethods: string[] = [];
for (const method of grpcStreamMethods) {
const descriptor: any = Reflect.getOwnPropertyDescriptor(constructor.prototype, method);
GrpcStreamMethod("PersonService", method)(constructor.prototype[method], method, descriptor);
}
};
}
export const PERSON_SERVICE_NAME = "PersonService";
So we can change the script, generate and update when run project
"start:dev": "npm run proto:gen && nest start --watch",
person module file
import { Module } from '@nestjs/common';
import { PersonController } from './person.controller';
import { PersonService } from './person.service';
import { APP_INTERCEPTOR } from '@nestjs/core';
import { GrpcLoggingInterceptor } from 'src/test.interceptor';
@Module({
controllers: [PersonController],
providers: [
PersonService,
{
provide: APP_INTERCEPTOR,
useClass: GrpcLoggingInterceptor,
},
],
})
export class PersonModule {}
person controller file
import { Controller } from '@nestjs/common';
import { PersonService } from './person.service';
import { Call, Metadata } from '@grpc/grpc-js';
import {
People,
Person,
PersonById,
PersonServiceControllerMethods,
} from 'src/proto/person';
@Controller('person')
@PersonServiceControllerMethods()
export class PersonController {
constructor(private readonly personService: PersonService) {}
findOne(data: PersonById, metadata: Metadata, call: Call): Person {
console.log('client access server controller', metadata, call);
return this.personService.findOne(data);
}
findAll(): People {
return this.personService.findAll();
}
}
we can see just use PersonServiceControllerMethods decorator imported from ts file, otherwise every function will need us add @GrpcMethod
person service
import { Injectable } from '@nestjs/common';
import {
People,
Person,
PersonById,
PersonServiceController,
} from 'src/proto/person';
@Injectable()
export class PersonService implements PersonServiceController {
private readonly people: Person[] = [
{ id: 1, name: 'Iron Man', power: 'Technology' },
{ id: 2, name: 'Spider Man', power: 'Spider Powers' },
{ id: 3, name: 'Thor', power: 'Thunder' },
];
findOne(request: PersonById): Person {
const person = this.people.find(({ id }) => id === request.id);
console.log('client calls server findOne function');
if (!person) {
throw new Error(`Person with id ${request.id} not found`);
}
return person;
}
findAll(): People {
return {
people: this.people,
};
}
}
register in app module
import { Module } from '@nestjs/common';
import { PersonModule } from './person/person.module';
@Module({
imports: [PersonModule],
})
export class AppModule {}
fill url and params in bloomrpc
run:

Run server successful
Achieve client
set script
add running dependency
pnpm add @nestjs/microservices
add dev dependency
pnpm add ts-proto -D
add script in package.json scripts
"proto:gen": "protoc --plugin=protoc-gen-ts_proto=./node_modules/.bin/protoc-gen-ts_proto --ts_proto_out=./proto/ --ts_proto_opt=nestJs=true src/proto/*.proto"
excute script
pnpm run proto
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
async function bootstrap() {
const app = await NestFactory.create(AppModule);
await app.listen(3001);
}
bootstrap();
to be easy, write the moudle in app.module directly
Here the url is server’s grpc url
register client module
// app.module.ts
import { Module } from '@nestjs/common';
import { ClientsModule, Transport } from '@nestjs/microservices';
import { join } from 'path';
import { GrpcClientService } from './client/client.service';
import { PersonController } from './client/client.controller';
@Module({
imports: [
ClientsModule.register([
{
name: 'PERSON_PACKAGE',
transport: Transport.GRPC,
options: {
package: 'person', // package name in proto file
protoPath: join(__dirname, '../../proto/person.proto'),
url: 'localhost:5000',
},
},
]),
],
controllers: [PersonController],
providers: [GrpcClientService],
})
export class AppModule {}
client controller
import { Controller, Get, Param } from '@nestjs/common';
import { GrpcClientService } from './client.service';
@Controller('person')
export class PersonController {
constructor(private readonly grpcClientService: GrpcClientService) {}
@Get(':id')
async getPersonById(@Param('id') id: string) {
const person = await this.grpcClientService
.findPersonById({ id: Number(id) })
.toPromise();
return person;
}
@Get()
async getAllPeople() {
const people = await this.grpcClientService.findAllPeople().toPromise();
return people;
}
}
client service
Importrant thing, can concern on how to call server function
// grpc-client.service.ts
import { Inject, Injectable, OnModuleInit } from '@nestjs/common';
import { ClientGrpc } from '@nestjs/microservices';
import { Observable } from 'rxjs';
import {
People,
Person,
PersonById,
PersonServiceClient,
} from 'src/proto/person';
@Injectable()
export class GrpcClientService implements OnModuleInit {
private personService: PersonServiceClient;
// inject grpc client service
constructor(@Inject('PERSON_PACKAGE') private client: ClientGrpc) {}
onModuleInit() {
// create grpc client when module initials
this.personService = this.client.getService('PersonService');
}
// call grpc server's findOne function
findPersonById(id: PersonById): Observable<Person> {
return this.personService.findOne(id);
}
// call grpc server's findMany function
findAllPeople(): Observable<People> {
return this.personService.findAll({});
}
}
this.client.getService()
create a proxy object which can :
- mapping service of server(define sevice in proto file)
- automaticly handle the details about grpc commication
- provide the same function interface as server has
when you excute getService('PersonService')
:
- can create a client object inluding
FindOne
andFindAll
function - These functions can be called directly like just call dev func
- low-level can handle the request and response of grpc
Test
access http://localhost:3001/person/1
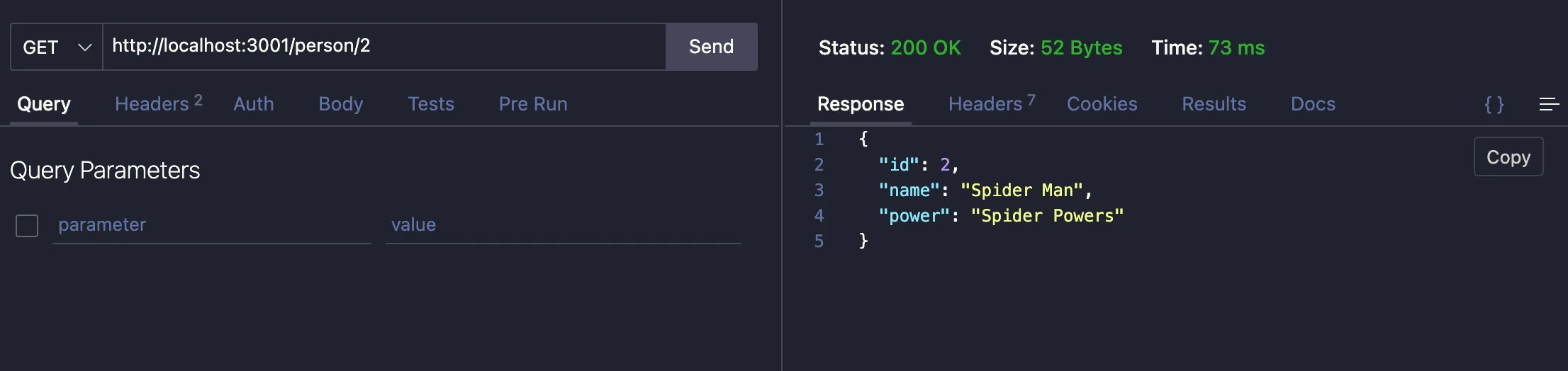
At the same time, print logs we added in controller and service
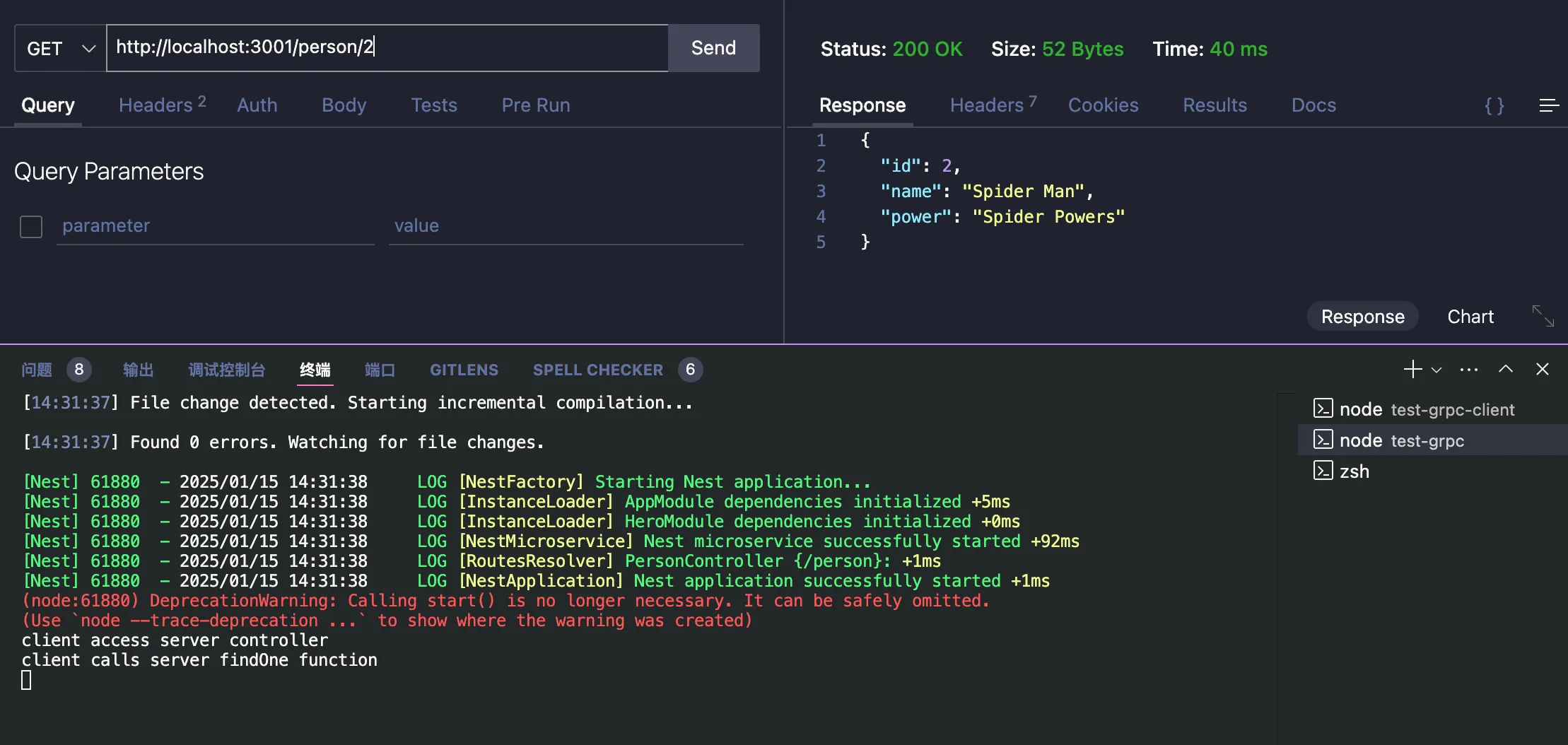
Attention
If you pay close attention, you might notice that there are two additional parameters in the server-side method, and their types come from @grpc/grpc-js
.
findOne(data: PersonById, metadata: Metadata, call: Call): Person {
console.log('client access server controller');
return this.personService.findOne(data);
}
So what’s the effect about metadata and call
metadata:
Metadata
type object, in order to transmit metadata data information of request- Common Uses:
- transmit access information like token
@GrpcMethod('PersonService', 'FindOne')
findOne(data: PersonById, metadata: Metadata) {
const token = metadata.get('authorization')[0]; // get token
// ... check logic
}
- transmit tracking ID:
const traceId = metadata.get('x-trace-id')[0];
- set custom header information:
metadata.set('custom-header', 'value');
call:
- represent current grpc call’s context information
- main function:
- get call state
@GrpcMethod()
findOne(data: PersonById, metadata: Metadata, call: ServerUnaryCall<any>) {
console.log(call.getPeer()); // Get client url
console.log(call.cancelled); // Check if the call was cancelled
}
- handle the events of steam method:
@GrpcStreamMethod()
streamPeople(data$: Observable<PersonById>, metadata: Metadata, call: ServerDuplexStream<any, any>) {
call.on('cancelled', () => {
// handle canceling call
console.log('Stream was cancelled');
});
}
- get setting information about request:
const settings = call.getSettings();
classic usage:
@Controller()
export class PersonController {
@GrpcMethod('PersonService')
async findOne(
data: PersonById,
metadata: Metadata,
call: ServerUnaryCall<any>
) {
// get access information
const token = metadata.get('authorization')[0];
// get origin of call
const clientAddress = call.getPeer();
// check call is canceled or not
if (call.cancelled) {
throw new Error('Call was cancelled');
}
// add response header
metadata.set('response-time', Date.now().toString());
return { /* people data */ };
}
}
So can use in these scenario:
- handle metadata about request(headers, access token …)
- control and monitor lifecycle of grpc call
- add log,monitor or other focus on
Attention please, not all functions need these two args.you can ignore them if you don’t need handle metadata or call context.