React 19 Unleashed: A Deep Dive into New Horizons
Introduction to React 19
React 19 marks a pivotal moment in the evolution of React, a library that has been integral to the frontend development world since its inception. Released on December 5, 2024, this version isn’t just an incremental update; it’s a significant leap forward, redefining how developers approach building user interfaces. With a strong emphasis on performance, developer experience, and modern web architecture, React 19 introduces a set of features that aim to streamline development processes while enhancing application efficiency.
The Philosophy Behind React 19
React has always been about simplifying UI development, and version 19 doubles down on this philosophy. The new release focuses on:
- Performance Optimization: By introducing tools like the React Compiler, React 19 addresses performance bottlenecks with less manual intervention from developers.
- Simplification: Making complex operations like form handling, asynchronous data management, and server-side rendering more intuitive.
- Interoperability: Enhancing how React works with other web technologies, including Web Components and server-side technologies.
- User Experience: Ensuring smoother, faster, and more responsive applications through new APIs and rendering strategies.
Key Features of React 19
1. React Compiler
The React Compiler is a groundbreaking feature that transforms the way React applications are built. It compiles React’s JSX into plain JavaScript, performing optimizations like automatic memoization, which was previously a manual process.
Code Example:
// Before React 19 (React 18 with manual optimization)
import { useMemo } from 'react';
function ExpensiveCalculation({ value }) {
const result = useMemo(() => {
let sum = 0;
for (let i = 0; i < 1000000; i++) {
sum += i;
}
return sum;
}, [value]);
return <div>Result: {result}</div>;
}
// After React 19 (No useMemo needed)
function ExpensiveCalculation({ value }) {
let sum = 0;
for (let i = 0; i < 1000000; i++) {
sum += i;
}
return <div>Result: {sum}</div>;
}
2. Actions
Actions in React 19 provide a new paradigm for handling user interactions, particularly in forms.
Code Example:
// Form submission with Actions
async function submitForm(formData) {
const response = await fetch('/api/submit', { method: 'POST', body: formData });
if (!response.ok) throw new Error('Failed to submit form');
return await response.json();
}
function FormComponent() {
return (
<form action={submitForm}>
<input type="text" name="name" placeholder="Enter name" />
<button type="submit">Submit</button>
</form>
);
}
3. Server Components
Server Components in React 19 are now a more mature feature, allowing for components to run on the server during the initial render. This approach:
Code Example:
// Server Component fetching data before rendering
import { Suspense } from 'react';
export default async function ServerComponent() {
const data = await fetchData();
return (
<Suspense fallback={<div>Loading...</div>}>
<ClientComponent data={data} />
</Suspense>
);
}
function ClientComponent({ data }) {
return <div>Data from server: {data.name}</div>;
}
// Assuming fetchData is an async function that fetches data from an API
async function fetchData() {
const response = await fetch('/api/data');
return response.json();
}
4. Enhanced Error Handling
React 19 improves how errors are reported and managed, particularly during hydration:
Code Example:
import { ErrorBoundary } from 'react-error-boundary';
function ErrorFallback({error}) {
return (
<div>
<p>Something went wrong:</p>
<pre>{error.message}</pre>
</div>
);
}
function MyComponent() {
// This might throw an error
throw new Error('Something went wrong');
}
export default function App() {
return (
<ErrorBoundary FallbackComponent={ErrorFallback}>
<MyComponent />
</ErrorBoundary>
);
}
5. Web Components Support
Integration with Web Components has been bolstered, allowing React applications to leverage the broader ecosystem of custom elements:
Code Example:
// Custom Web Component
class MyCustomElement extends HTMLElement {
connectedCallback() {
this.innerHTML = '<div>This is a custom element!</div>';
}
}
customElements.define('my-custom-element', MyCustomElement);
// React Component using the Web Component
function ReactWrapper() {
return <my-custom-element />;
}
export default ReactWrapper;
6. Asset Loading and Management
New APIs for managing how assets are loaded:
Code Example:
import { preload } from 'react-dom';
function App() {
// Preload an image before rendering
preload('/path/to/image.jpg', { as: 'image' });
return (
<div>
<img src="/path/to/image.jpg" alt="Preloaded Image" />
</div>
);
}
export default App;
7. New Hooks
New hooks simplify common tasks:
- use() for handling promises:
// React 19 - Using the use() hook for asynchronous operations
import { use } from 'react';
function FetchData() {
const data = use(fetchDataFromServer());
return data ? <div>Data: {data.name}</div> : <div>Loading...</div>;
}
async function fetchDataFromServer() {
const response = await fetch('/api/data');
return response.json();
}
- useFormStatus() for form handling:
// React 19 - Form status management with useFormStatus
import { useFormStatus } from 'react-dom';
function SubmitButton() {
const { pending } = useFormStatus();
return <button type="submit" disabled={pending}>Submit</button>;
}
- useOptimistic() for optimistic UI updates:
// React 19 - Optimistic UI updates with useOptimistic
import { useOptimistic } from 'react';
function LikeButton({ likes }) {
const [optimisticLikes, addOptimisticLike] = useOptimistic(likes, (state, amount) => state + amount);
async function handleLike() {
addOptimisticLike(1);
await fetch('/api/like', { method: 'POST' });
}
return <button onClick={handleLike}>Likes: {optimisticLikes}</button>;
}
React 19 vs. React 18 - A Detailed Comparison
- Performance: React 19’s compiler brings automatic performance optimizations, whereas React 18 required more manual tuning.
- Development Experience: The new hooks (use(), useFormStatus(), useOptimistic()) in React 19 simplify common patterns, making development more intuitive than in React 18.
- Server-Side Rendering: Enhanced server components in React 19 offer more out-of-the-box solutions for SSR compared to the experimental features in React 18.
- Error Handling: React 19’s error handling is more sophisticated, offering a better developer experience.
Conclusion
React 19 is more than just an upgrade; it’s a reimagining of how React can work to make web development more efficient, enjoyable, and performant. For developers transitioning from React 18, the learning curve might include understanding the new paradigms like Actions and the Compiler. However, the benefits of this version are clear: a cleaner code base, enhanced performance, and a smoother development process. As always, the React community will play a crucial role in refining these features through feedback and shared experiences.
For those eager to dive into React 19, start with small, non-critical components of your applications, allowing you to get accustomed to the new patterns and benefits without risking larger projects’ stability.
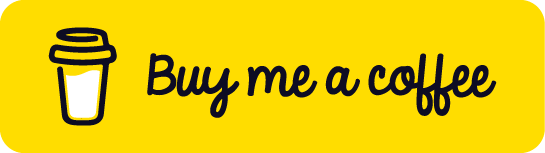